Download the tutorial code here: helloworldservice.zip
Lets start with building a simple windows service in Visual Studio which will write "Hello World" text with current Date and Time stamp into a text file.
- Open Visual Studio
- Navigate to "File -> New -> Project" menu option
- Navigate to "Visual C# -> Windows Desktop" under installed templates options
- Select "Windows Service"
- Enter "Project Name" and "Location" as shown below
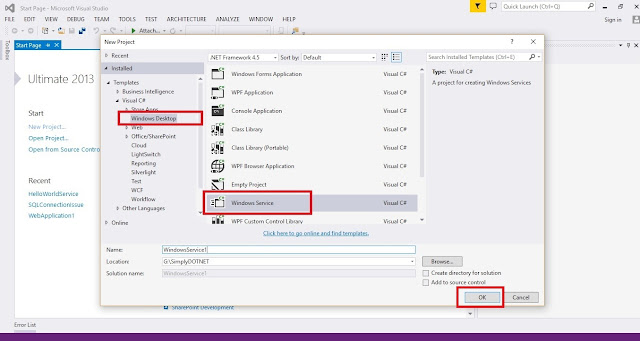
- Rename the "Service.cs" file to "HelloWorldService.cs"
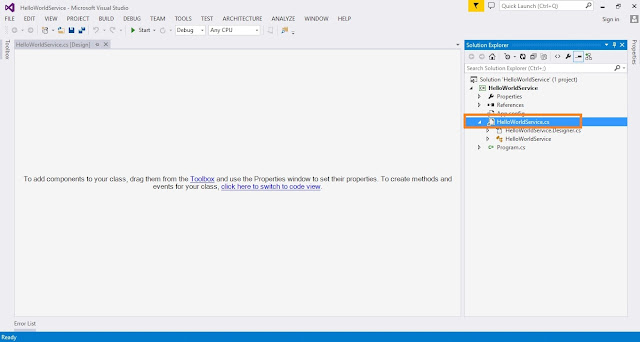
- Go to code behind file of "HelloWorldService.cs" file and enter the following code:
/// <summary>
/// Create instance of timer class and set the interval.
/// define the timer elapsed event
/// call the start method of the timer object
/// </summary>
public HelloWorldService()
{
InitializeComponent();
Timer oTimer = new Timer();
oTimer.Elapsed += oTimer_Elapsed;
oTimer.Interval = 6000;
oTimer.Start();
}
/// <summary>
/// define the logic to write the file with "HelloWorld" text with datetime stamp
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
void oTimer_Elapsed(object sender, ElapsedEventArgs e)
{
StreamWriter oStreamWriter = new StreamWriter("G:\\SimplyDOTNET\\ServiceOutput\\HelloWorld.txt", true);
oStreamWriter.WriteLine("Hello World" + DateTime.Now.ToString());
oStreamWriter.Close();
oStreamWriter = null;
}
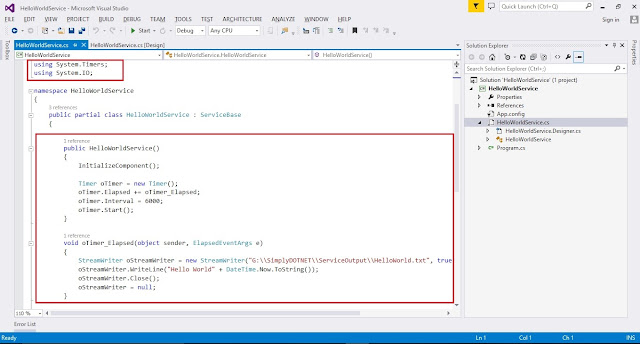
- run the service
- donot close the alert window which is shown
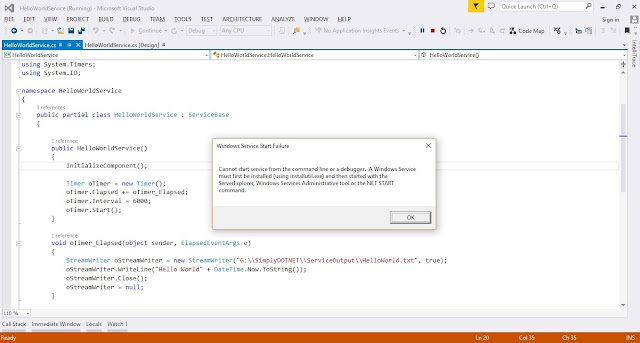
- navigate to the folder which is set as a destination to write the text file
- you can find a file and if you open it, you will find the text is written to the file
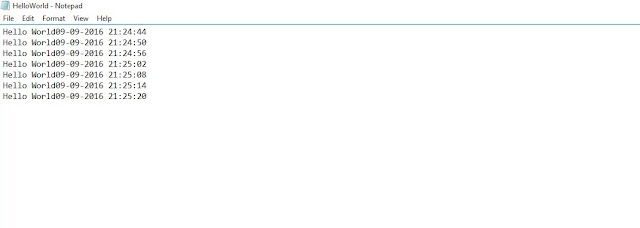
Hope this helps.
Comments
Post a Comment